How to convert your webcam into a smart security camera using Python & OpenCV
In this project-tutorial, we will be learning how to covert a webcam into a smart security camera using Python and OpenCV. In this project, will be able to read vision from the webcam camera, detect unusual movement and finally alert the owner by playing a warning sound after detection of movement.
Requirements:
1 – Python 3.0 and above
2 – PyCharm IDE or Visual Studio Code IDE or Anaconda (Jupyter Notebook) or any other IDE of your choice.
This project builds upon what we covered in the previous tutorial where we introduced what OpenCV is and we also differentiated between Face detection and Face recognition incase you’re wondering what’s the distinction between the two. The previous project-tutorial can be found here;
Face detection using Python and OpenCV
For this tutorial, we shall follow the steps below;
Step-1: Install and Import the required libraries
Create a new python file in your IDE and give it a name of your choice. The two main library packages that we will be using for this project are OpenCV and winsound. Unlike OpenCV, winsound library comes pre-installed with Python, so we need to install OpenCV and import it as shown below;
Run the code snippet below in the Terminal window of your IDE or in a new cell of Anaconda (Jupyter Notebook) to install OpenCV.
pip install opencv-python
To import the library, just simply write this line of code.
# Import the opencv library.
import cv2
Step-2: Capture video from Webcam
Here, we will begin by ensuring that we’re able to capture a video using the webcam.
# import the required library
import cv2
# capture the video-feed from the webcam
cam = cv2.VideoCapture(0)
while(True):
# the read method that reads whatever is happening on the webcam
ret, frame = cam.read()
# display the webcam video feed
cv2.imshow('Webcam', frame)
# break the loop when 'q' is pressed.
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cam.release()
cv2.destroyAllWindows()
Step-3: Get the difference between two frames.
This is used to identify and detect movement if there is any motion, for this, we will need to add the following code to the while loop. Upon running this code, you will see that if you don’t move, there is no difference and nothing will be shown on the screen, it will be completely dark. You will only see motion on the camera if there is movement, that is if there is a difference in the frames.
ret, frame1 = cam.read()
ret, frame2 = cam.read()
diff = cv2.absdiff(frame1, frame2)
Step-4: Convert to grayscale
If you noticed, the captured video above is colored and reading colorful video frames might result into an error. so to turn that video frame difference to grayscale, we will need the following line of code that will be added to the while loop.
gray = cv2.cvtColor(diff, cv2.COLOR_RGB2GRAY)
Step-5: Covert to blur, create a threshold & dilate.
Here we convert the video frame to blur (gaussian blur) by providing the size and the sigma index. Upon running the blur code, you will notice the difference in the video frame from the previous video.
Then we need to create a threshold, this is important because it helps us to get rid of the noise. Here we add the threshold values are 20 (minimum) and 255 (this being the maximum) and the threshold type. Upon running the threshold code, you will notice the video is sharper and brighter.
Then we need to create a dilation, this is the opposite of threshold. Here the dilation will be done three times.
# convert to blur
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# create a threshold
_, thresh = cv2.threshold(blur, 20, 255, cv2.THRESH_BINARY)
# dilation - opposite of threshold
dilated = cv2.dilate(thresh, None, iterations=3)
Step-6: Create contours
Contours basically create boarders onto the detected objects in motion. Here we create two variables one called “contours” and another one we don’t really care, we’ll just use a dash. We will then find all contours available on the screen then declare the methods used as shown in the code below.
contours, _ = cv2.findContours(dilated, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
cv2.drawContours(frame1, contours, -1, (0, 255, 0), 2)
Step-7: Create a rectangular boarder on the moving subject
You noticed that the above code doesn’t create a rectangular boarder on to the moving subject, but rather captures all moving parts of the body. To create a rectangular border onto the moving subjects, we will need a for loop as shown below;
for c in contours:
if cv2.contourArea(c) < 5000:
continue
x, y, w, h = cv2.boundingRect(c)
cv2.rectangle(frame1, (x, y), (x+w, y+h), (255, 0, 0), 3)
In the above code, inside the for-loop, we create a condition that if the contour area is smaller than a certain value, we continue to skip this (ignore small animals like dogs, cats etc) and show the rest of the stuff. Then we go ahead and declare the x, y, w and h values to get the dimensions of the contours on the detected subject. We then add a color and also specify the thickness as shown in the code above.
Step-8: Add an alert sound
Here we add an alerting sound that will notify the owner of the premises in case of an intruder.
import winsound
winsound.Beep(500, 200)
winsound.PlaySound('intruder_alert_alarm.mp3', winsound.SND_ASYNC)
The full code
# import the required libraries
import cv2
import winsound
# capture the video-feed from the webcam
cam = cv2.VideoCapture(0)
while(True):
# use the read method to capture the video frames
ret, frame1 = cam.read()
ret, frame2 = cam.read()
diff = cv2.absdiff(frame1, frame2)
# convert to gray color
gray = cv2.cvtColor(diff, cv2.COLOR_RGB2GRAY)
# convert to blur
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# create a threshold
_, thresh = cv2.threshold(blur, 20, 255, cv2.THRESH_BINARY)
# create a dilation - opposite of threshold
dilated = cv2.dilate(thresh, None, iterations=3)
# create contours
contours, _ = cv2.findContours(dilated, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
#cv2.drawContours(frame1, contours, -1, (0, 255, 0), 2)
#The above line doesn't give a rectangular boarder, so to do this, we...
#create a for-loop
for c in contours:
if cv2.contourArea(c) < 5000:
continue
x, y, w, h = cv2.boundingRect(c)
cv2.rectangle(frame1, (x, y), (x+w, y+h), (255, 0, 0), 3)
winsound.Beep(500, 200)
winsound.PlaySound('intruder_alert_alarm.mp3', winsound.SND_ASYNC)
# display the webcam video feed
cv2.imshow('Webcam', frame1)
# break the loop when 'q' is pressed.
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cam.release()
cv2.destroyAllWindows()
Video output from the Smart Webcam with alert alarm
References:
[1]. OpenCV website: https://opencv.org/about/
[2]. OpenCV Documentation: https://docs.opencv.org/4.x/d9/df8/tutorial_root.html
If you liked this project-tutorial, please subscribe to our YouTube Channel for more D.I.Y tutorials and projects.
Leave a comment down below incase you have any concerns…
Follow us on our different social media platforms;- LinkedIn, Facebook, Instagram, TikTok, & Pinterest.
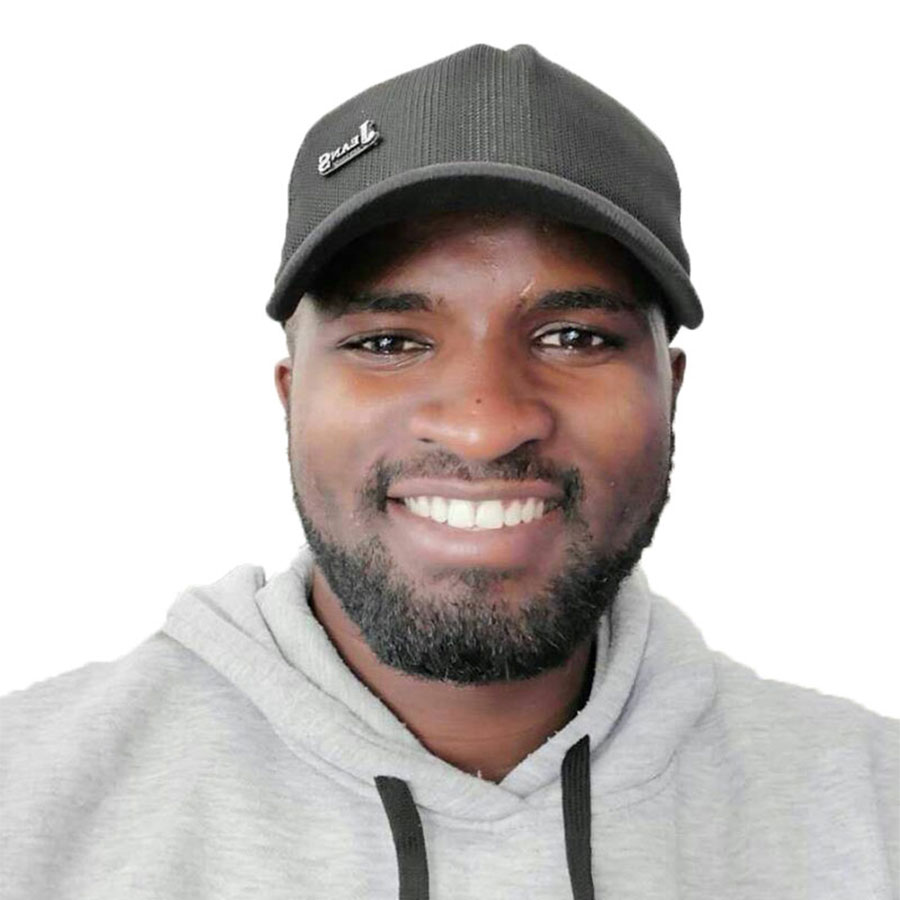
About the Author…
Tumusiime Kwiringira a.k.a Tum, is an embedded systems engineer, D.I.Y Life Hacker, Tech Blogger, YouTuber, Founder and Lead-Engineer at sonalabs.org. He’s passionate about innovation and building D.I.Y projects to inspire the young generation in the fields of Mechatronics and Artificial Intelligence… Read more…