How to build a Scientific Calculator using Python
In this project-tutorial, we will be learning how to build our own Scientific Calculator using Python to perform simple mathematical operations.
Requirements:
1 – Python 3.0 and above
2 – PyCharm IDE or Visual Studio Code IDE or Anaconda (Jupyter Notebook) or any other IDE of your choice.
For this tutorial, we shall follow the steps below;
Step-1: Import the required libraries
Create a new python file in your IDE and give it a name of your choice. The main library packages that we will be using for this project are tkinter, math. Both of these libraries come pre-installed with Python, all we need to do is to import them as shown in the code below;
from tkinter import *
import math
About the required Libraries
- tkinter library
Tkinter is an acronym for “Tk interface”. Tk was developed as a GUI extension for the Tcl scripting language. Tkinter or Tk interface is Python’s de-facto standard GUI (Graphical User Interface) package and is included in all standard Python Distributions. In fact, it’s the only framework built into the Python standard library.
- math library
The math library is a built-in module in the Python 3 standard library that provides standard mathematical constants and function. It can be used to perform various mathematical calculations, such as numeric, trigonometric, logarithmic, and exponential calculations.
Step-2: Create the GUI Window
For this project, we will begin by creating a blank GUI window. To do this, we write the code below and in this code.
from tkinter import *
root=Tk()
root.mainloop()
In the above code, the first line means we import all classes and methods from the tkinter library module. The class that makes the GUI window is the Tk() class inside the tkinter library module and this is assigned to the object root. So upon running this code with just the two lines, we will not see anything, so in order to be able to see the GUI we need to add another line of code that keeps the GUI window open always until it’s closed by using the “mainloop()” method which is inside the Tk() class onto the object “root”. It should be noted that every other code(s) will be written between the two lines of code that is; between “root=Tk()” and “root.mainloop()”.
Step-3: Set title, add icon, images, background, and geometry.
In the next step, we will give the application a title, add an icon, set the desired background color plus also set its geometry and make it un-resizable.
To achieve this, we need to use the following lines of code. Ensure to have the image of the icon and other images you intend to use in your project folder.
#Add title, bg color, & set geometry
root.title('Scientific Calculator')
root.config(bg='green4')
icon=PhotoImage(file = 'calculator.png')
root.iconphoto(False, icon)
root.geometry('662x410')
root.resizable(False, False)
Then the other thing, we will be adding two other images to the GUI window (root) that is; the calculator image on the left side and the SonaLabs logo Image on the right side and in order to achieve this, we use the following lines of code below. These images need to be added to the project folder otherwise you will get an error in the code.
#Logo Image - calculator image
logoImage = PhotoImage(file='calc.png')
logoLabel = Label(root, image=logoImage, bg='green4')
logoLabel.grid(row=0,column=0)
#Logo Image - sonalabs image
logoImage1 = PhotoImage(file='sona.png')
logoLabel1 = Label(root, image=logoImage1, bg='green4')
logoLabel1.grid(row=0,column=7)
Step-4: Create an Entry field on the GUI window
In this step we will be creating an entry field that will be capturing our input values. We achieve this by using the 2 lines of code below. The first line of code here is about setting parameters for the entry field and the other is for aligning the entry field on the GUI window.
#Create an entry field on to the window.
entryField=Entry(root, font=('arial',20,'bold'), bg='#ffffff', fg='BLACK', bd=10,relief=SUNKEN, width=30, justify='right')
entryField.grid(row=0,column=0,columnspan=8,padx=0,pady=5)
Step-5: Create a list of the calculator buttons to be used
In this step we will be creating a list of the buttons that are needed on our calculator and to achieve this, we will use the code below and in this code, you will notice that in order to implement buttons for square root, cube root, x power y, x power 3, x power 2 , and division, we use characters special such as “chr(8731), “x\u02b8”, “x\u00B3”, “x\u00B2” and “chr(247)” as shown in the code below.
#Create a list of the buttons to be used
button_text_list = ["cosθ", "tanθ", "sinθ", "π", "C", "CE", "√", "+",
"cosh", "tanh", "sinh", "2π", "1", "2", "3", "-",
chr(8731), "x\u02b8", "x\u00B3", "x\u00B2", "4", "5", "6", "x",
"In", "deg", "rad", "e", "7", "8", "9", "chr(247)",
"log10", "(", ")", "x!", "0", ".", "%", "="]
Step-6: Create a for loop for the button list above
In this step, we will begin by creating two variables (rowValue and columnValue) to store the row and column values respectively. Then we will create a for loop for the button list above and here, we will be adding different buttons in the value of i and we will incrementing the columnValue by one and if the columnValue is greater than index 7, then we will move to a new row while maintaining the same column as shown in the code below.
#Create variables to store row and column values
rowValue=2
columnValue=0
#Create a for loop for the button list above
for i in button_text_list:
#Add buttons to the calculator
button=Button(root, width=6,height=2,relief=SUNKEN,text=i,bg='green4',fg='white',font=('arial',15,'bold'),activebackground='#fff', command=lambda button=i: click(button))
button.grid(row=rowValue,column=columnValue,pady=1,padx=0)
columnValue+=1
if columnValue>7:
rowValue+=1
columnValue=0
Step-7: Adding functionality to the buttons of the calculator.
The final step is to add functionality to our calculator buttons that is to ensure that when a user clicks on a button, it is able to perform its intended functionality. Here we will begin by creating a function “def click(value)” and within this function, we will create a variable “ent” to capture and store the entered value and then we will create a variable “answer” and declare it as null. Then we will use “if statements” for every button beginning with the “clear” button within a “try-pass” block. Add the functionality code for each and every button as shown in the code below.
#Adding functionality to the buttons of the calculator.
def click(value):
ent = entryField.get() # Capture the entered value
answer = '' # Declare the variable answer as null
try:
# The C (clear-one by one) button...
if value == 'C':
ent=ent[0:len(ent)-1] # Reduce the length of any given entry by one
entryField.delete(0,END) # delete everything first
entryField.insert(0,ent) # then insert
return
# The C (clear-all) button...
elif value == 'CE':
entryField.delete(0,END)
# The Sqaure-root button...
elif value == '√':
answer=math.sqrt(eval(ent))
# The pi button...
elif value == 'π':
answer=math.pi
# Add functionality code for other buttons here...
entryField.delete(0,END)
entryField.insert(0,answer)
except SyntaxError:
pass
The Full Code
Below is the full python code for the Scientific Calculator. Feel free to alter the code to your liking.
from tkinter import *
import math
#Adding functionality to the buttons of the calculator.
def click(value):
ent = entryField.get() # Capture the entered value
answer = '' # Declare the variable answer as null
try:
# The C (clear-one by one) button...
if value == 'C':
ent=ent[0:len(ent)-1] # Reduce the length of any given entry by one
entryField.delete(0,END) # delete everything first
entryField.insert(0,ent) # then insert
return
# The C (clear-all) button...
elif value == 'CE':
entryField.delete(0,END)
# The Sqaure-root button...
elif value == '√':
answer=math.sqrt(eval(ent))
# The pi button...
elif value == 'π':
answer=math.pi
# The 2pi button...
elif value == '2π':
answer=2*math.pi
# The cosθ button...
elif value == 'cosθ':
answer=math.cos(math.radians(eval(ent)))
# The tanθ button...
elif value == 'tanθ':
answer=math.tan(math.radians(eval(ent)))
# The sinθ button...
elif value == 'sinθ':
answer=math.sin(math.radians(eval(ent)))
# The cosh button...
elif value == 'cosh':
answer=math.cosh(eval(ent))
# The tanh button...
elif value == 'tanh':
answer=math.tanh(eval(ent))
# The sinh button..
elif value == 'sinh':
answer=math.sinh(eval(ent))
# The cube root button
elif value == 'chr(8731)':
answer=eval(ent)**(1/3)
# The X power Y button
elif value == 'x\u02b8':
entryField.insert(END,'**')
return
# The X power 3 button
elif value == 'x\u00B3':
answer=eval(ent) ** 3
# The X power 2 button
elif value == 'x\u00B2':
answer=eval(ent) ** 2
# Log to the base e (In) button...
elif value == 'In':
answer=math.log2(eval(ent))
# Degree button...
elif value == 'deg':
answer=math.degrees(eval(ent))
# Radian button...
elif value == 'rad':
answer=math.radians(eval(ent))
# The e-value button...
elif value == 'e':
answer=math.e
# Log10 button...
elif value == 'log10':
answer=math.log10(eval(ent))
# The factorial button
elif value == 'x!':
answer=math.factorial(ent)
# The division button
elif value == 'chr(247)':
entryField.insert(END, "/")
return
# The Equal Button
elif value == '=':
answer=eval(ent)
else:
# Insert the numeric values
entryField.insert(END,value)
return
entryField.delete(0,END)
entryField.insert(0,answer)
except SyntaxError:
pass
root=Tk()
#Add title, bg color, & set geometry
root.title('Scientific Calculator')
root.config(bg='green4')
icon=PhotoImage(file = 'calculator.png')
root.iconphoto(False, icon)
root.geometry('662x410')
root.resizable(False, False)
#Logo Image 1
logoImage = PhotoImage(file='calc.png')
logoLabel = Label(root, image=logoImage, bg='green4')
logoLabel.grid(row=0,column=0)
#Logo Image 2
logoImage1 = PhotoImage(file='sona.png')
logoLabel1 = Label(root, image=logoImage1, bg='green4')
logoLabel1.grid(row=0,column=7)
#Create an entry filed on to the window.
entryField=Entry(root, font=('arial',20,'bold'), bg='#ffffff', fg='BLACK', bd=10,relief=SUNKEN, width=30, justify='right')
entryField.grid(row=0,column=0,columnspan=8,padx=0,pady=5)
#Create a list of the buttons to be used
button_text_list = ["cosθ", "tanθ", "sinθ", "π", "C", "CE", "√", "+",
"cosh", "tanh", "sinh", "2π", "1", "2", "3", "-",
chr(8731), "x\u02b8", "x\u00B3", "x\u00B2", "4", "5", "6", "x",
"In", "deg", "rad", "e", "7", "8", "9", chr(247),
"log10", "(", ")", "x!", "0", ".", "%", "="]
#Create variables to store row and column values
rowValue=2
columnValue=0
#Create a for loop for the button list above
for i in button_text_list:
#Add buttons to the calculator
button=Button(root, width=6,height=2,relief=SUNKEN,text=i,bg='green4',fg='white',font=('arial',15,'bold'),activebackground='#fff', command=lambda button=i: click(button))
button.grid(row=rowValue,column=columnValue,pady=1,padx=0)
columnValue+=1
if columnValue>7:
rowValue+=1
columnValue=0
root.mainloop()
References:
[1]. tkinter library documentation: https://docs.python.org/3/library/tk.html
[2]. math library documentation: https://docs.python.org/3/library/math.html
If you liked this project-tutorial, please subscribe to our YouTube Channel for more D.I.Y tutorials and projects.
Leave a comment down below incase you have any concerns…
Follow us on our different social media platforms;- LinkedIn, Facebook, Instagram, TikTok, & Pinterest.
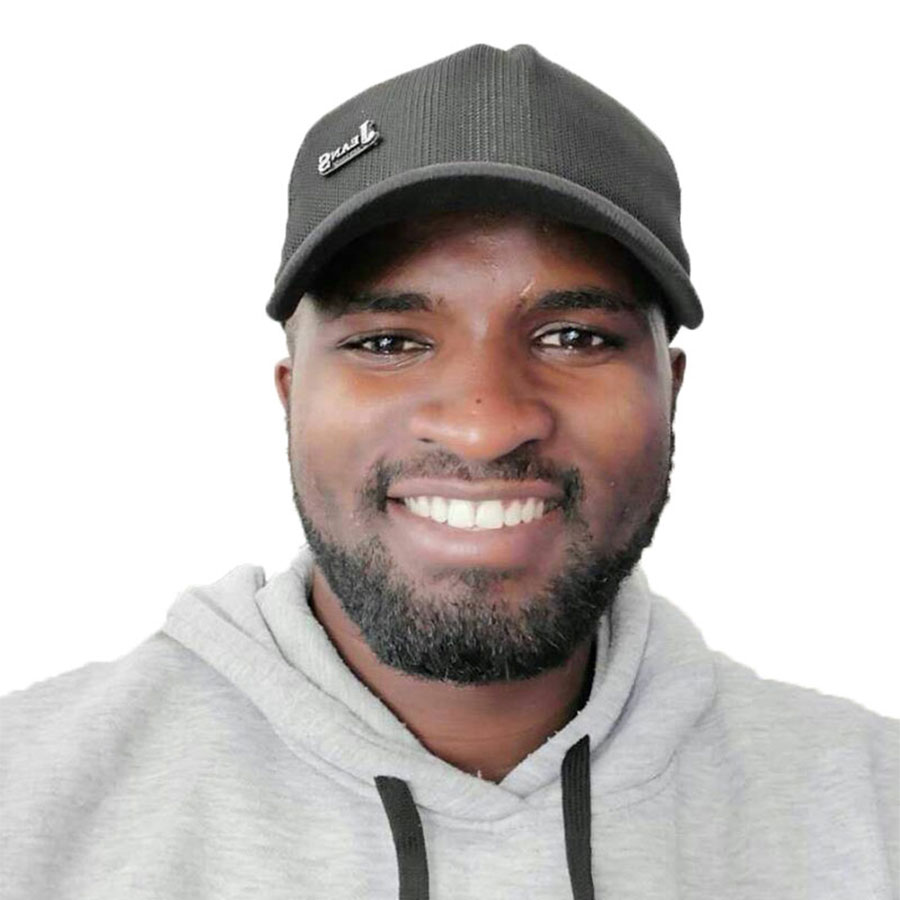
About the Author…
Tumusiime Kwiringira a.k.a Tum, is an embedded systems engineer, D.I.Y Life Hacker, Tech Blogger, YouTuber, Founder and Lead-Engineer at sonalabs.org. He’s passionate about innovation and building D.I.Y projects to inspire the young generation in the fields of Mechatronics and Artificial Intelligence… Read more…