How to Build your own ‘ALEXA’ using Python
In this Project-tutorial, we are going to learn how to build our own Digital Virtual Assistant (D.I.Y Alexa) that functions like Alexa, Siri, Google Assistant, or Cortana using a few lines of code in Python. Our virtual assistant will be capable of performing simple tasks such as telling the current time, playing YouTube videos, making internet searches, providing weather updates, telling jokes, opening specific web pages, opening and closing of Windows operating system applications and other simple tasks.
Requirements:
1 – Python 3.0 and above
2 – PyCharm IDE or Visual Studio Code IDE or any other IDE of your choice
Step-1: Install the required packages:
The required packages for our digital virtual assistant are listed below;
1. pyttsx3 (Text to Speech)
This python library package enables text-to-speech conversion. Its is compatible with both Python 2 and 3 plus it works offline and it supports multiple TTS engines including Sapi5, nsss and espeak.
To install this pyttsx3 package, run the following command in the Terminal window of your IDE
pip install pyttsx3
This Library is used for performing speech recognition with support for several engines and APIs, and it’s capable of operating online and offline.
To install this package, run the following command in the Terminal window of your IDE
pip install SpeechRecognition
3. wikipedia
This python library makes it easy to access and parse data from Wikipedia. It searches wikipedia, gets article summaries, gets data links and images from a page and more.
To install wikipedia, run the following command in the Terminal window of your IDE
pip install wikipedia
4. DateTime
This python package provides a DateTime data type.
To install DateTime, run the following command in the Terminal window of your IDE
pip install DateTime
5. Webbrowser
The webbrowser python module provides a high-level interface to allow displaying of web-based documents to users.
To install webbrowser, run the following command in the Terminal window of your IDE
pip install webbrowser
6. Pyjokes
This python package, provides online jokes for programmers (jokes as a service)
To install pyjokes, run the following command in the Terminal window of your IDE
pip install pyjokes
7. Pywhatkit
This python library is a Simple and Powerful WhatsApp and YouTube Automation Library with many useful Features
To install pywhatkit, run the following command in the Terminal window of your IDE
pip install pywhatkit
8. Pyaudio
PyAudio provides Python bindings for PortAudio v19, the cross-platform audio I/O library. With PyAudio, you can easily use Python to play and record audio on a variety of platforms, such as GNU/Linux, Microsoft Windows, and Apple macOS.
To install pyaudio, run the following command in the Terminal window of your IDE
pip install pyaudio
Step-2: Testing the Speech Recognition:
The next step involves testing to see if our DIY Alexa is capable of recognizing our speech and for this we begin by importing the required libraries that is; the pyttsx3 (text to speech library) and the speech recognition library as shown in the code below; So in the code below, we begin by creating a listener to recognize our voice, we then use a try-except block because there are times when the microphone that is used as a source might not work else we pass. We then create a variable “voice” that stores the speech recognizer that is called to listen to the source. We then used the “command” variable whose value is the google API that converts the voice to text. Here you can use any other API of your choice. As an example we set an if statement where if the word Alexa is mentioned in our speech command, the command will be printed as shown below.
import pyttsx3
import speech_recognition as sr
listener = sr.Recognizer()
try:
with sr.Microphone() as source:
print('Listening...')
voice = listener.listen(source)
command = listener.recognize_google(voice)
command = command.lower()
if 'alexa' in command:
print(command)
except:
pass
Output:
Upon first trial, we said “Alexa you are the best I love you” and because the word Alexa was included, she was able to print what we said. If you don’t mention her name Alexa in the command, nothing will be printed.
Listening...
Alexa you are the best I love you
Process finished with exit code 0
Step-3: Using text to speech – making Alexa speak to us:
The next step is to make Alexa talk to us what we instruct her to say. In this step, we will be using the pyttsx3 (text to speech) library. So here we begin by creating an engine to speak to us and we initialize this engine. Then we add the instructions for Alexa to say to us before we give her instructions and then we set the engine to run and await.
import pyttsx3
engine = pyttsx3.init()
engine.say('Hello there, iam Alexa, your desktop assistant')
engine.say('what can i do for you?')
engine.runAndWait()
Step-4: Changing Alexa’s voice to a female voice and adjusting the rate of speed:
In this step, upon running the code above, you will notice that by default Alexa has a male voice and we need to change that to a feminine lovely voice with a proper rate of speed of her voice. To do this, we use the four lines of code shown below;
#selecting a female voice
voices = engine.getProperty('voices')
engine.setProperty('voice', voices[1].id)
#adjusting the rate of speed of Alexa's voice
rate = engine.getProperty('rate')
engine.setProperty('rate', 115)
Step-5: Making Alexa speak what we say – she hates being called ‘Siri’:
In this step, we will be making Alexa to speak out what we tell to say or talk. So to achieve this, we will create a function “alexa_talk” that will be called if the word “Alexa” is said in the command. Otherwise if you call her Siri, she will correct you as shown in the code below. Also we will also need to replace the Alexa so that it doesn’t appear in the print out was she captures our commands. This is done by one line code put between the if statements below.
def alexa_talk(text):
engine.say(text)
engine.runAndWait()
if 'alexa' in command:
alexa_talk(command)
command = command.replace('alexa', '')
if 'siri' in command:
alexa_talk('My name is Alexa not Siri, do not call me that')
Step-6: Make Alexa play music on YouTube
Before we do this, we will have to first of all put the entire try-except block within a new function ” def accept_command” and we will return command as shown below.
def accept_command():
try:
.....
except:
pass
return command
So to make Alexa play music, we will have to create another function called “def play_alexa():” and we will begin by a print test by printing out “Playing” just as an indication that the song is about to be played and then finally we will call the function as shown in the code below.
def play_alexa():
command = accept_command()
print(command)
if 'play' in command:
print('playing')
alexa_talk('playing')
play_alexa()
Upon running this code and saying “Alexa play Ed Sheeran”, the output will be printed as shown below, the word Alexa will not be included because we replaced it with space earlier on.
Listening...
play ed sheeran
Playing
As we can see in the output above, we also need to get rid of the word “play” in the output, as we just want Alexa to start playing the song directly. We achieve this by altering the code above (the play_alexa() function) as shown below;
command = command.replace('play', '')
talk('playing' + song)
print(song)
Finally, so to be able to make Alexa play songs directly from YouTube, we will need the library called Pywhatkit. Since it was earlier installed, we just need to import it and modify the play-song function code a little bit. Here we will alter the code by removing the print part and replacing it as shown below.
import pywhatkit
pywhatkit.playonyt(song) # yt means YouTube
When the play_alexa() function code is modified, it should look like this below. Upon running this code, wait for Alexa to introduce herself, she will print out “Listening…” and then tell her which song you want to be played on YouTube. She will open up your default web browser, go to the YouTube website and play your song as instructed.
def play_alexa():
command = accept_command()
#print(command)
if 'play' in command:
song = command.replace('play', '')
alexa_talk('playing' + song)
pywhatkit.playonyt(song)
play_alexa()
Step-7: Make Alexa tell the time
In order to make Alexa tell us the time, we shall have to use the “datetime” library module which was already installed, we just have to import it. Then we shall modify the “play_alexa()” function code above to add the functionality of telling time. So in the code below, upon saying the word “time” in your command, it will be captured by Alexa and then the current time will be fetched and shown in a desired string format (either in a 12 hour system or a 24 hour system depending on the set format). We can also print it out on the screen if we wish.
import datetime
elif 'time' in command
time = datetime.datetime.now().strftime('%I:%M %p')
print(time)
alexa_talk('The current time is ' + time)
Step-8: Search on Wikipedia
So to be able to make Alexa search for content on Wikipedia, we shall have to use the “wikipedia” library module which was already installed, we just have to import it. Then we shall still modify the “play_alexa()” function code above to add the functionality of searching on Wikipedia.
import wikipedia
elif 'wikipedia' in command:
content = command.replace('wikipedia ', '')
info = wikipedia.summary(person, 4)
print(info)
Step-9: Make Alexa tell jokes
Just like other functionalities, to do this, we shall have to use the “pyjokes” library module which was already installed, we just have to import it. Then we shall still modify the “play_alexa()” function code above to add the functionality of making Alexa tell jokes. We can also add a line of code that allows Alexa to ask us to repeat again what we’ve said to her in case she didn’t capture the command well.
#Alexa telling jokes
elif 'joke' in command:
alexa_talk(pyjokes.get_joke())
else:
alexa_talk('I did not catch that, please say the command again...')
Step-10: Make Alexa active all the time
To do this, we shall have to create a while loop and add the last line of code “play_alexa()” within that loop as shown below. This way, you don’t have to run the code again and again.
while True:
play_alexa()
It should be noted that so many functionalities can be added to Alexa that we did not include in this tutorial. Feel free to alter it to your liking.
The Full Code:
Below is the full code for creating your own D.I.Y Alexa.
import datetime
import pyttsx3
import speech_recognition as sr
import pywhatkit
import wikipedia
import pyjokes
listener = sr.Recognizer()
engine = pyttsx3.init()
#chnaging to a female voice
voices = engine.getProperty('voices')
engine.setProperty('voice', voices[1].id)
#adjusting the rate of speed of the speaker engine
rate = engine.getProperty('rate')
engine.setProperty('rate', 150)
def alexa_talk(text):
engine.say(text)
engine.runAndWait()
def accept_command():
try:
with sr.Microphone() as source:
alexa_talk('Hello there, iam Alexa, your desktop assistant')
alexa_talk('How may i help you?')
print('Listening...')
voice = listener.listen(source)
command = listener.recognize_google(voice)
command = command.lower()
if 'alexa' in command:
alexa_talk(command)
command = command.replace('alexa', '')
if 'siri' in command:
alexa_talk('My name is Alexa not Siri, do not call me that')
except:
pass
return command
def play_alexa():
command = accept_command()
#print(command)
#Alexa playing video on YouTube
if 'play' in command:
song = command.replace('play', '')
alexa_talk('playing' + song)
pywhatkit.playonyt(song)
#Alexa telling the time
elif 'time' in command:
time = datetime.datetime.now().strftime('%I:%M %p')
print(time)
alexa_talk('The current time is ' + time)
#ALexa searching for stuff on wikipedia
elif 'wikipedia'+'who is' + 'tell me about' in command:
content = command.replace('wikipedia ', '')
info = wikipedia.summary(content, 2)
print(info)
alexa_talk(info)
#Alexa telling jokes
elif 'joke' in command:
alexa_talk(pyjokes.get_joke())
else:
alexa_talk('I did not catch that, please say the command again...')
while True:
play_alexa()
Conclusion:
So basically, this is how you can create your own Virtual Assistant (D.I.Y Alexa) using python that is capable of performing simple tasks such as telling the current time, playing YouTube videos, making internet searches, and so much more… Hope you had fun…
References:
[1]. Pyttsx3 (Text-to-Speech) Documentation: https://pyttsx3.readthedocs.io/en/latest/
[2]. Speech Recognition Documentation: https://github.com/Uberi/speech_recognition#readme
[3]. Datetime Documentation: https://docs.python.org/3/library/datetime.html
[4]. Webbrowser Documentation: https://docs.python.org/3/library/webbrowser.html
[5]. Pywhatkit Documentation: https://github.com/Ankit404butfound/PyWhatKit/wiki
[6]. Pyaudio Documentation: https://people.csail.mit.edu/hubert/pyaudio/docs/
[7]. Wikipedia Documentation: https://wikipedia.readthedocs.io/en/latest/
If you liked this project-tutorial, please subscribe to our YouTube Channel for more D.I.Y tutorials and projects.
Leave a comment down below incase you have any concerns…
Follow us on our different platforms such as LinkedIn, Facebook, Instagram, TikTok, Pinterest & GitHub.
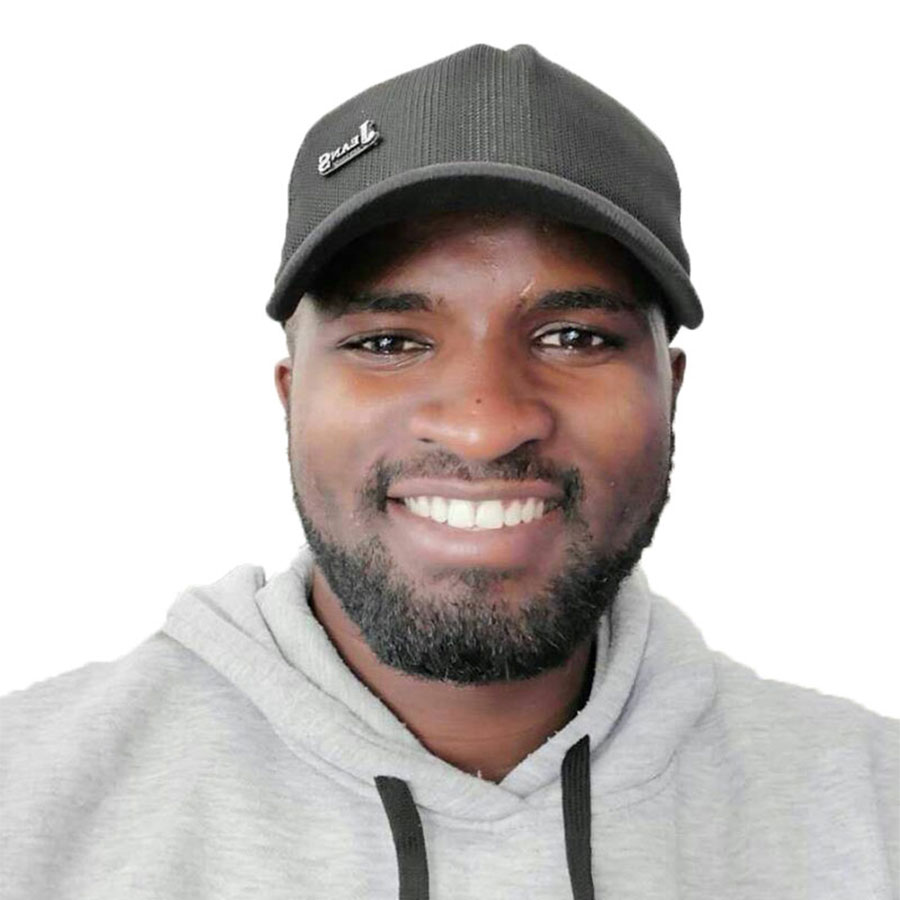
About the Author…
Tumusiime Kwiringira a.k.a Tum, is an embedded systems engineer, D.I.Y Life Hacker, Tech Blogger, YouTuber, Founder and Lead-Engineer at sonalabs.org. He’s passionate about innovation and building D.I.Y projects to inspire the young generation in the fields of Mechatronics and Artificial Intelligence… Read more…